1. Add Dependency
We use the jsonrpc4j library, the latest version is 1.5.3 https://github.com/briandilley/jsonrpc4j2. Create AutoJsonRpcServiceImplExporter
3. Create ServerAPI interface
4. Create ServerAPI implementation
5. Deploy to glassfish server
6. Use Postman to test API
6.1. Test 'multiplier' method
Send POST request with 'application/json' to endpoint 'http://localhost:8080/jsonrpc4j/api'6.2. Test 'printPerson' method
6.3. Test 'printPersons' method
Send POST request with 'application/json' to endpoint 'http://localhost:8080/jsonrpc4j/api'Download Source Code
7. Implement your own JSON RCP Client
It is quite simple to write a controller to invoke JSON-RPC API by using JsonRpcRestClient Download JSON RCP Client Source Code8. Deploy JSON RCP Client and Test
8.1. Deploy JSON RPC Client
'SpringJsonRPCClient' can be deployed in the same Glassfish with package 'SpringJsonRPCExample'
8.2. Test JSON RPC Client
Enter the appropriated urls to web browser to invoke API
http://localhost:8080//jsonrpc4j-client/multiplier?a=1111111&b=1111111
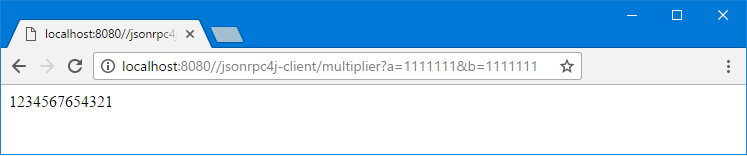
http://localhost:8080//jsonrpc4j-client/printperson
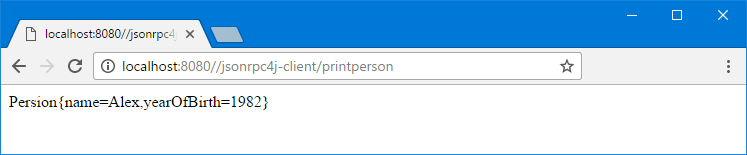
http://localhost:8080//jsonrpc4j-client/printpersons
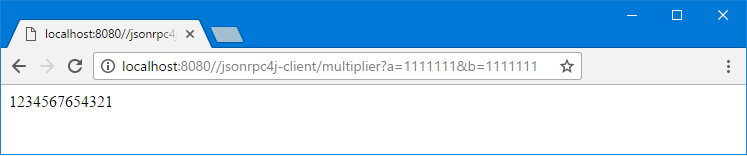
No comments :
Post a Comment